Point of Sales
Point Of Sales
Tampilan Blue J :
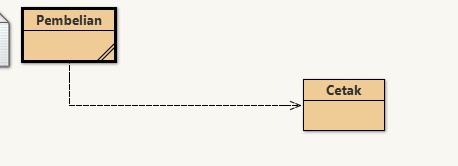
Class Pembelian :
import javafx.application.Application;
import javafx.application.Platform;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.stage.Stage;
import javafx.scene.layout.StackPane;
import javafx.collections.*;
import javafx.scene.paint.*;
import javafx.scene.text.*;
import javafx.scene.Group;
import java.util.Random;
public class Pembelian extends Application
{
Text textKasir = new Text("Kasir :");
Text KodeBarang = new Text("");
Text textHarga = new Text("");
Text textJumlah = new Text("");
Text textTotalBayar = new Text("");
Text NamaBarang = new Text("");
Text HargaBarang = new Text("");
Text TotalHargaText = new Text("");
TextField textFieldJumlah = new TextField ();
ObservableList<String> optionsKasir = FXCollections.observableArrayList(
"Gusrai",
"Mono",
"Jonmin"
);
final ComboBox comboBoxKasir = new ComboBox(optionsKasir);
ObservableList<String> optionsBarang = FXCollections.observableArrayList(
"CS123",
"LC001",
"BL001"
);
final ComboBox comboBoxBarang = new ComboBox(optionsBarang);
private int TotalHarga;
private int harga;
@Override
public void start(Stage stage) throws Exception
{
Scene scene = new Scene(new Group(), 500, 250);
stage.setTitle("Pembelian");
textKasir.setFont(Font.font("SanSerif",18));
KodeBarang.setFont(Font.font("SanSerif",18));
textHarga.setFont(Font.font("SanSerif",18));
textJumlah.setFont(Font.font("SanSerif",18));
textTotalBayar.setFont(Font.font("SanSerif",18));
GridPane grid = new GridPane();
grid.setVgap(10);
grid.setHgap(10);
grid.setPadding(new Insets(10, 10, 10, 10));
grid.add(new Label("Kasir: "), 0, 0);
grid.add(comboBoxKasir, 1,0);
grid.add(new Label("Kode Barang: "), 0, 1);
grid.add(comboBoxBarang, 1,1);
EventHandler<ActionEvent> event =
new EventHandler<ActionEvent>() {
public void handle(ActionEvent e)
{
if(comboBoxBarang.getValue() == "CS123")
{
NamaBarang.setText("Malboro Black Filter");
HargaBarang.setText("Rp12000");
harga = 12000;
}
else if(comboBoxBarang.getValue() == "LC001")
{
NamaBarang.setText("Malboro Merah");
HargaBarang.setText("Rp28000");
harga = 28000;
}
else if(comboBoxBarang.getValue() == "BL001")
{
NamaBarang.setText("Malboro Ice Burst");
HargaBarang.setText("Rp30000");
harga = 30000;
}
}
};
comboBoxBarang.setOnAction(event);
grid.add(new Label("Nama Barang: "), 0, 2);
grid.add(NamaBarang, 1,2);
grid.add(new Label("Harga: "), 0, 3);
grid.add(HargaBarang, 1,3);
grid.add(new Label("Jumlah: "), 0, 4);
grid.add(textFieldJumlah, 1,4);
EventHandler<ActionEvent> eventJumlah = new EventHandler<ActionEvent>() {
public void handle(ActionEvent e)
{
TotalHarga = Integer.parseInt(textFieldJumlah.getText()) * harga;
TotalHargaText.setText("Rp"+Integer.toString(TotalHarga));
}
};
textFieldJumlah.setOnAction(eventJumlah);
grid.add(new Label("Total Bayar: "), 0, 5);
grid.add(TotalHargaText, 1,5);
Text title=new Text("Hello Fortune Teller");
title.setFont(Font.font("SanSerif",36));
Button button = new Button("Cetak");
grid.add(button, 1,6);
button.setOnAction(this::buttonClick);
Group root = (Group)scene.getRoot();
root.getChildren().add(grid);
stage.setScene(scene);
stage.show();
}
private void buttonClick(ActionEvent event)
{
Cetak c = new Cetak(NamaBarang, textFieldJumlah.getText(), TotalHargaText);
c.showCetak();
}
}
Class Cetak :
import javafx.application.Application;
import javafx.application.Platform;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.stage.Stage;
import javafx.scene.layout.StackPane;
import javafx.collections.*;
import javafx.scene.paint.*;
import javafx.scene.text.*;
import javafx.scene.Group;
import java.util.Random;
public class Cetak
{
private Text NamaBarang = new Text(""), TotalHargaText = new Text("");
private String jumlahBeli;
public Cetak(Text namaBarang, String jumlah, Text TotalHarga)
{
NamaBarang = namaBarang;
TotalHargaText = TotalHarga;
jumlahBeli = jumlah;
}
public void showCetak()
{
Stage stage = new Stage();
Scene scene = new Scene(new Group(), 500, 250);
stage.setTitle("Nota");
GridPane grid = new GridPane();
grid.setVgap(10);
grid.setHgap(15);
grid.setPadding(new Insets(10, 10, 10, 10));
grid.add(new Label("Pembelian"),0,0);
grid.add(NamaBarang,0,1);
grid.add(new Label(jumlahBeli),1,1);
grid.add(new Label("Harga"),0,2);
grid.add(TotalHargaText,1,2);
Group root = (Group)scene.getRoot();
root.getChildren().add(grid);
stage.setScene(scene);
stage.show();
}
}
INPUT
OUTPUT
Tampilan Blue J :
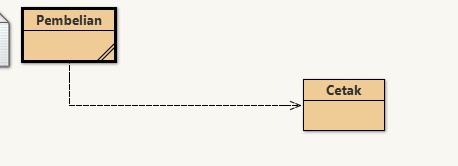
Class Pembelian :
import javafx.application.Application;
import javafx.application.Platform;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.stage.Stage;
import javafx.scene.layout.StackPane;
import javafx.collections.*;
import javafx.scene.paint.*;
import javafx.scene.text.*;
import javafx.scene.Group;
import java.util.Random;
public class Pembelian extends Application
{
Text textKasir = new Text("Kasir :");
Text KodeBarang = new Text("");
Text textHarga = new Text("");
Text textJumlah = new Text("");
Text textTotalBayar = new Text("");
Text NamaBarang = new Text("");
Text HargaBarang = new Text("");
Text TotalHargaText = new Text("");
TextField textFieldJumlah = new TextField ();
ObservableList<String> optionsKasir = FXCollections.observableArrayList(
"Gusrai",
"Mono",
"Jonmin"
);
final ComboBox comboBoxKasir = new ComboBox(optionsKasir);
ObservableList<String> optionsBarang = FXCollections.observableArrayList(
"CS123",
"LC001",
"BL001"
);
final ComboBox comboBoxBarang = new ComboBox(optionsBarang);
private int TotalHarga;
private int harga;
@Override
public void start(Stage stage) throws Exception
{
Scene scene = new Scene(new Group(), 500, 250);
stage.setTitle("Pembelian");
textKasir.setFont(Font.font("SanSerif",18));
KodeBarang.setFont(Font.font("SanSerif",18));
textHarga.setFont(Font.font("SanSerif",18));
textJumlah.setFont(Font.font("SanSerif",18));
textTotalBayar.setFont(Font.font("SanSerif",18));
GridPane grid = new GridPane();
grid.setVgap(10);
grid.setHgap(10);
grid.setPadding(new Insets(10, 10, 10, 10));
grid.add(new Label("Kasir: "), 0, 0);
grid.add(comboBoxKasir, 1,0);
grid.add(new Label("Kode Barang: "), 0, 1);
grid.add(comboBoxBarang, 1,1);
EventHandler<ActionEvent> event =
new EventHandler<ActionEvent>() {
public void handle(ActionEvent e)
{
if(comboBoxBarang.getValue() == "CS123")
{
NamaBarang.setText("Malboro Black Filter");
HargaBarang.setText("Rp12000");
harga = 12000;
}
else if(comboBoxBarang.getValue() == "LC001")
{
NamaBarang.setText("Malboro Merah");
HargaBarang.setText("Rp28000");
harga = 28000;
}
else if(comboBoxBarang.getValue() == "BL001")
{
NamaBarang.setText("Malboro Ice Burst");
HargaBarang.setText("Rp30000");
harga = 30000;
}
}
};
comboBoxBarang.setOnAction(event);
grid.add(new Label("Nama Barang: "), 0, 2);
grid.add(NamaBarang, 1,2);
grid.add(new Label("Harga: "), 0, 3);
grid.add(HargaBarang, 1,3);
grid.add(new Label("Jumlah: "), 0, 4);
grid.add(textFieldJumlah, 1,4);
EventHandler<ActionEvent> eventJumlah = new EventHandler<ActionEvent>() {
public void handle(ActionEvent e)
{
TotalHarga = Integer.parseInt(textFieldJumlah.getText()) * harga;
TotalHargaText.setText("Rp"+Integer.toString(TotalHarga));
}
};
textFieldJumlah.setOnAction(eventJumlah);
grid.add(new Label("Total Bayar: "), 0, 5);
grid.add(TotalHargaText, 1,5);
Text title=new Text("Hello Fortune Teller");
title.setFont(Font.font("SanSerif",36));
Button button = new Button("Cetak");
grid.add(button, 1,6);
button.setOnAction(this::buttonClick);
Group root = (Group)scene.getRoot();
root.getChildren().add(grid);
stage.setScene(scene);
stage.show();
}
private void buttonClick(ActionEvent event)
{
Cetak c = new Cetak(NamaBarang, textFieldJumlah.getText(), TotalHargaText);
c.showCetak();
}
}
Class Cetak :
import javafx.application.Application;
import javafx.application.Platform;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.layout.*;
import javafx.scene.text.Font;
import javafx.scene.text.Text;
import javafx.stage.Stage;
import javafx.scene.layout.StackPane;
import javafx.collections.*;
import javafx.scene.paint.*;
import javafx.scene.text.*;
import javafx.scene.Group;
import java.util.Random;
public class Cetak
{
private Text NamaBarang = new Text(""), TotalHargaText = new Text("");
private String jumlahBeli;
public Cetak(Text namaBarang, String jumlah, Text TotalHarga)
{
NamaBarang = namaBarang;
TotalHargaText = TotalHarga;
jumlahBeli = jumlah;
}
public void showCetak()
{
Stage stage = new Stage();
Scene scene = new Scene(new Group(), 500, 250);
stage.setTitle("Nota");
GridPane grid = new GridPane();
grid.setVgap(10);
grid.setHgap(15);
grid.setPadding(new Insets(10, 10, 10, 10));
grid.add(new Label("Pembelian"),0,0);
grid.add(NamaBarang,0,1);
grid.add(new Label(jumlahBeli),1,1);
grid.add(new Label("Harga"),0,2);
grid.add(TotalHargaText,1,2);
Group root = (Group)scene.getRoot();
root.getChildren().add(grid);
stage.setScene(scene);
stage.show();
}
}
INPUT
OUTPUT
Comments
Post a Comment